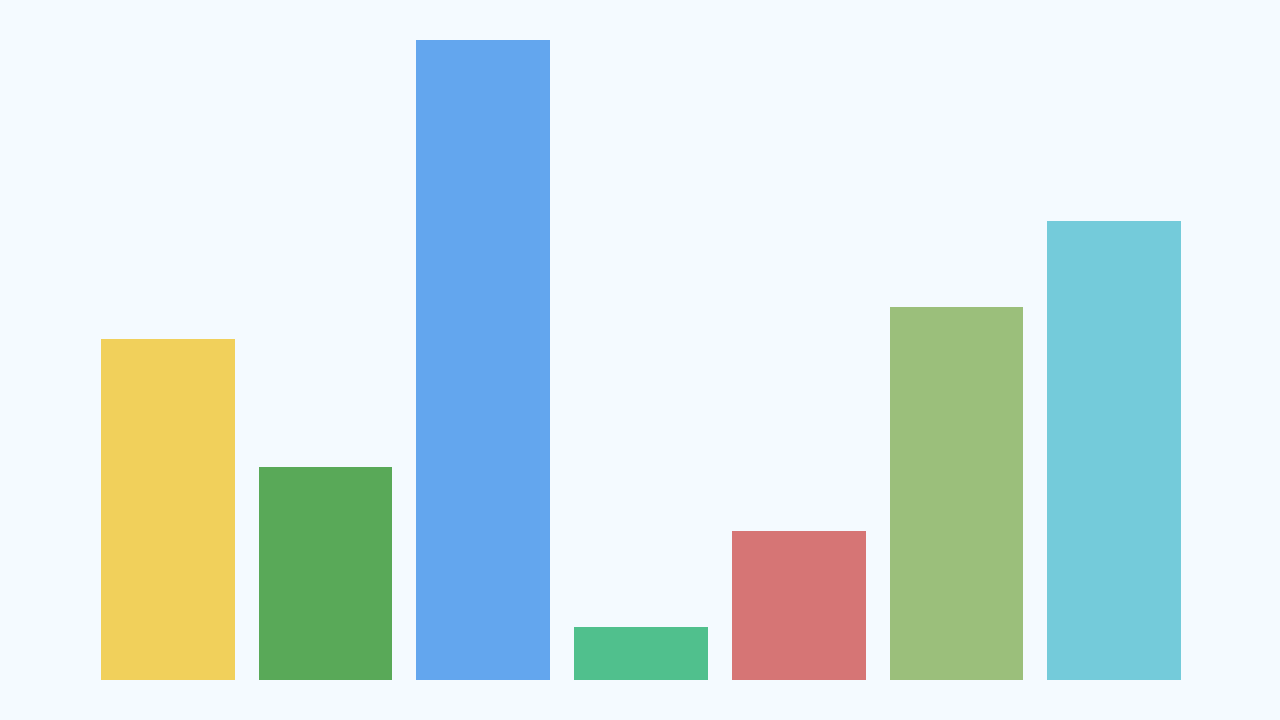
Bar Chart

Component that visualizes a dataset by rendering bars with lengths proportional to their corresponding values.
This interview requires you to write and execute code, building a simple interactive user interface using vanilla JavaScript or React, and styling it with CSS.
Component that visualizes a dataset by rendering bars with lengths proportional to their corresponding values.
Component that allows users to rate items by selecting a number of stars out of a maximum value.
Build a Traffic Light with Pure JavaScript: Red, Yellow, Green, and Beyond! 🚦
Build a tab component in React with a focus on accessibility and user experience.
A component that provides cities suggestions as the user types in an input field.
Component displays a list of selectable options when clicked and returns the selected value to the parent component.
Todo list enables users to seamlessly add, edit, delete tasks, and mark completion for efficient task management.
Application that allows users to perform basic arithmetic operations, such as addition, subtraction, multiplication, and division.
Interactive Tic Tac Toe game interface, allowing users to play against each other and displaying the winner or a draw.
A transfer list is a UI component for moving items between two lists, often used to manage and organize multiple selections.
Component that displays hierarchical data and allows users to expand or collapse nodes interactively.
Vertically stacked set of interactive headings that each reveal an associated section of content.
Nested commenting system that visually distinguishes between comment levels, akin to Reddit or Twitter discussions.
Interactive grid where users toggle node colors. Upon activating all nodes, the system automatically reverts them sequentially to their initial state.
Component that visually represents the percentage of a task's completion using a progress bar.
Component that tracks elapsed time with precision, providing start/stop/reset functionality and lap recording.
A Toast system in React shows temporary notifications of various types, allowing users to create and dismiss them individually.
Simulates dealing a hand of cards from a standard deck, displaying the drawn cards to the user.
Component that simulates a basic elevator system.
Reusable React component that formats a phone number input field in real-time.
Efficiently process and filter financial transactions based on a provided query.
A user interface displaying a list of products with a name-based filter, enhanced by styling and animation.
Wordle is a five-letter word puzzle with color-coded feedback for each guess, green (correct), yellow (misplaced), and gray (absent).
Generate a hierarchical table of contents from a HTML document.
React components for efficiently rendering large lists and tabular data
A dynamic interface where users draw two circles that change color when they intersect, offering real-time visual feedback on overlap.
React game component where players match country names with their corresponding capitals by clicking buttons.
A web component for users to input and submit a purchase value with real-time validation and feedback.
The component should accept a configuration prop that determines the type of transformation to apply.
Create a matrix of random colors and more
Beep boop! Program a virtual Roomba in this hands-on coding challenge
Video chat room using React Hooks, incorporating features such as a lobby, a list of participants, and the chat room itself.