How does the box model work in CSS?
Box model
Although it may not seem obvious when viewing a web page, every element in HTML can be considered a rectangular box.
Say your page contains a heading using the <h1/>
element.
That's a rectangular box.
The paragraph of text beneath it, that's a rectangular box.
And if those two elements are wrapped inside a <div/>
, well, that means your two smaller boxes are inside one larger box.
Calculating the size of each of these boxes requires more than just measuring the width and height of the content.
The box model consists of four distinct layers that determine the total size of an element:
- content: this is the core where your actual content lives, like text or images. The size of this area is directly influenced by your content or explicitly set through width and height properties.
- padding: this is what I like to call the 'breathing room' inside the box. It creates space between your content and the border. A key point that often comes up in development: padding is inside the box, and if your element has a background color, the padding will share that color.
- border: think of it as the outline of your box. While borders are typically hidden by default, they can be styled in various ways with different thicknesses, colors, and styles.
- margin: this is the space outside the box that separates it from other elements. A common interview question actually addresses the difference between margin and padding: margin creates space outside the box, while padding creates space inside it.
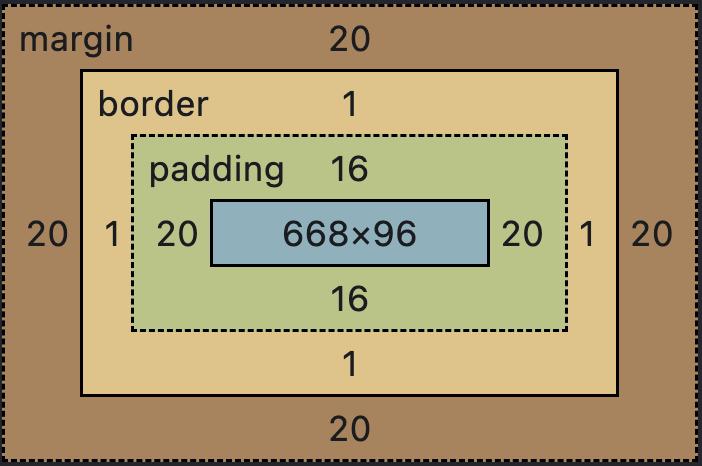
An important detail to understand is that by default, when you set a width/height on an element, it only controls the content box. The actual space the element takes up will be:
- Total width = content width + left padding + right padding + left border + right border + left margin + right margin
- Total height = content height + top padding + bottom padding + top border + bottom border + top margin + bottom margin
This is why many developers use box-sizing: border-box
in their CSS resets. It makes the width property include padding and border, which leads to more predictable layouts.
.box {
/* Makes width/height include content, padding, and border */
box-sizing: border-box;
}
With box-sizing: border-box
, the width and height you set include the content, padding, and border (but not margin).
- Total width = specified width + left margin + right margin
- Total height = specified height + top margin + bottom margin
You can run this code snippet in console of any website to see that every element is a box.
const allElements = document.querySelectorAll('*');
allElements.forEach(element => element.style.border = '1px solid tomato');